First of all, will see how this application works! Then will focus on the code behind the application.
Prerequisites to test the application
- Apache Tomcat installed.
- Maven installed.
- Configure SSL on Apache Tomcat.
- Configure Tomcat and Maven to deploy Maven projects to Tomcat.
- You are done! Get the source code and play with it!
How it works?
Points to consider
· We don’t use the database here. Therefore the credentials (Username & Passwords) are hardcoded.
· Application developed using Maven with Java and deployed to Apache Tomcat server.
· Tomcat server is configured with SSL certificate and it’s work on both
· Therefore this application works on both
http://localhost:8080/login-app (Unsecured connection) and
https://localhost:8443/login-app (Secured connection)
· Understand the basics of cookies
In our application, we can set the optional flags as we want.
Optional flags in the cookies are,
o Secure Flag
o HttpOnly Flag
What is a secure flag?
It is an option (additional flag) that can be set by the server when sending a new cookie to the user within an HTTP response. Normally cookies are transmitted in clear text. Therefore it can be observed by unauthorized parties. The purpose of the secure flag is to prevent cookie form being observed by unauthorized parties.
If the secure flag is set (true) for the cookie, it will not be transmitted through the unsecured connection.
i.e. Cookie will not be transmitted through HTTP connections. It will be transmitted through https connections.
Default value: false - Cookie will be transmitted through any type of connections.
If set to true – Cookie will be transmitted only through secured connections.
What is httpOnly flag?
It is also an option (additional flag) that can be set by the server when sending a new cookie to the user within an HTTP response.
The purpose of the httpOnly flag is to mitigate the risk of client side script (ex: JavaScript) accessing the protected cookie.
If the httpOnly flag is set (true) for the cookie, client-side scripts (JavaScript) cannot access the cookie.
Default value: false – JavaScript can access the cookie.
If set to true – JavaScript cannot access the cookie.
Now, will run the application!

Since the cookie is not created for the user, you’ll be automatically redirected to the login page. (Cookie will be created once the user logged in successfully).

Now we’ll have to consider 4 different scenarios as follows.
Secure Flag
|
httpOnly Flag
|
|
1.
|
False
|
False
|
2.
|
False
|
True
|
3.
|
True
|
False
|
4.
|
True
|
True
|
Username: abc
Password: 123
1. Secure flag is not set & httpOnly flag is not set
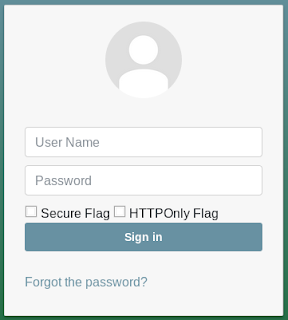
Once you signed in with the given credentials, the cookie will be created successfully.
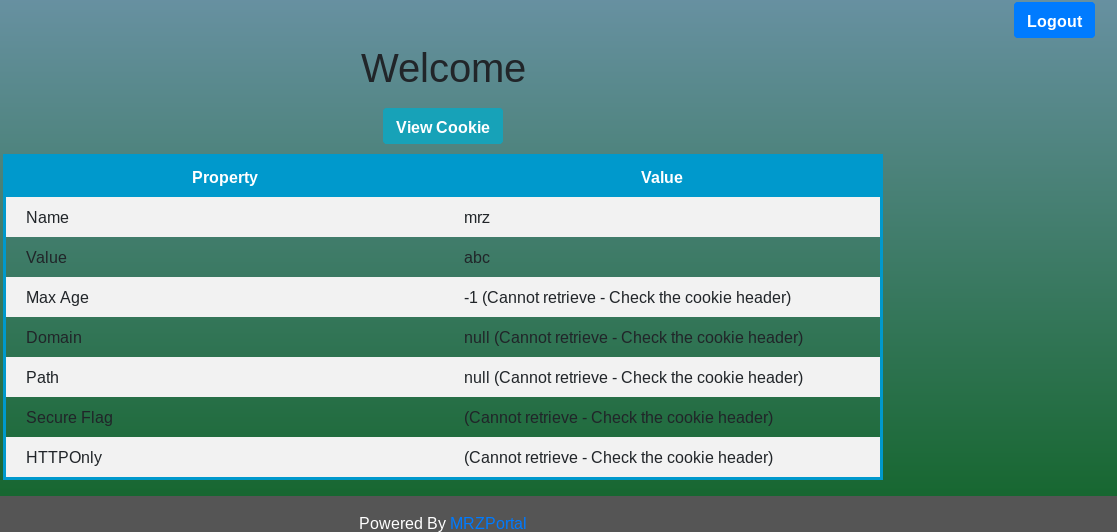
As the picture shown above, attributes will not be shown except the cookie name and value. You’ll have to check it through some cookie managers such as Cookies Manager+.
If you click on View Cookie button, you’ll see the output as follows. It is invoking a JavaScript function which is,
<script>
function show_cookie() {
alert(document.cookie);
}
</script>
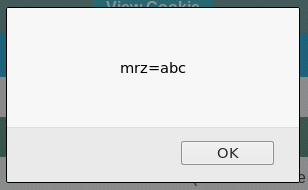
If you view the cookie through Cookie Manager+,
2. Secure flag is not set & httpOnly flag is set
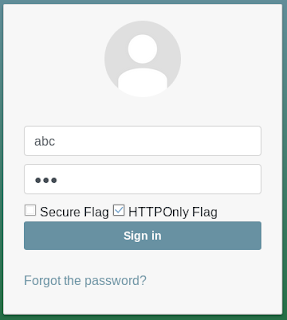
The httpOnly flag is set in this case. Therefore if you click on View Cookie button, you’ll see an empty alert box, since document.cookie will return null because JavaScript cannot access the cookie.
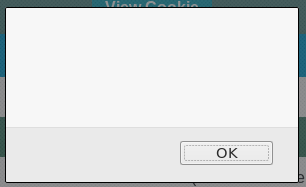
If you view the cookie through Cookie Manager+,
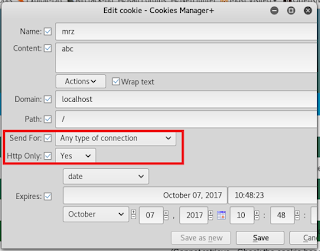
3. Secure flag is set & httpOnly flag is not set
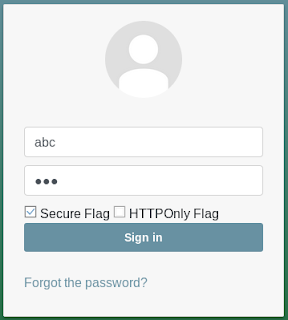
Secure flag is set in this case. Therefore cookie will not be transmitted through unsecured connections. Therefore cookie will not be created and the user will not be able to sign in.
Therefore will access the login application through https secure connection.

Now the cookie will be created and you’ll be able to sign in. Since the httpOnly flag is not set, View Cookie button will show the alert as usual. If you view the cookie through Cookie Manager+,
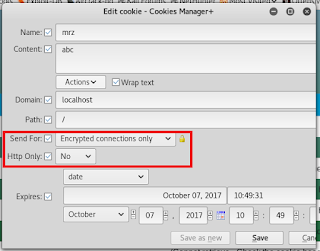
4. Both Secure flag & httpOnly flag is set
In this case, the cookie will be transmitted only through secured connections and JavaScript cannot access the cookie.
If you view the cookie through Cookie Manager+,
Code behind the application
Login form
We have 2 pages in the application.
1. login.jsp
2. index.jsp (Home Page)
1. First will have look on login.jsp
Login form
<form method="POST"> <input type="text" name="username" placeholder="User Name" required autofocus> <input type="password" name="password" placeholder="Password" required> <div> <label> <input type="checkbox" name="secure_flag" value="secure"> Secure Flag <input type="checkbox" name="httponly_flag" value="httponly"> HTTPOnly Flag </label> </div> <button name ="submit" type="submit">Sign in</button> </form>
When the user clicked on "Sign in" button
<% //When the user clicked on "sign in" button
if(request.getParameter("submit") != null)
{
String username = request.getParameter("username");
String password = request.getParameter("password");
String secure = request.getParameter("secure_flag");
String httponly = request.getParameter("httponly_flag");
//Validate the username and password
if ("abc".equals(username) && "123".equals(password))
{
//Create the cookie named "mrz" with the value of the username provided by the user
Cookie uname = new Cookie("mrz", request.getParameter("username"));
//Set the maximum age in seconds
uname.setMaxAge(60*60); //valid for 1 hour
//Set the domain for the cookie for which the domains are valid for the cookie
uname.setDomain("localhost");
//Set the path for the cookie.
uname.setPath("/"); //Cookie is valid for all the pages of localhost. boolean secure_stat = false;
boolean httponly_stat = false;
//If the user checked "Secure Flag"
if(secure != null)
secure_stat = true;
//If the user checked "httpOnly Flag"
if(httponly != null)
httponly_stat = true;
//Set the flags
uname.setSecure(secure_stat);
uname.setHttpOnly(httponly_stat);
//Add the cookie
response.addCookie(uname);
//Redirect the user to the home page
response.sendRedirect("/login-app");
}
else
{
out.println("Failed");
}
}
%>
2. Now will understand the index.jsp (Home page)
<%
//Get all the cookies and store it in the array named "cookies" Cookie[] cookies = request.getCookies();
boolean login_status = false;
if(cookies != null)
{
for (Cookie cookie : cookies)
{
if (cookie.getName().equals("mrz"))
{
login_status = true; //Load the table
}
}
//If the "mrz" cookie is not there, redirect back the user to login page.//Therefore user will not be able to view the home page without sign in.
if(!login_status)
{
response.sendRedirect("login.jsp");
}
}
else
{
response.sendRedirect("login.jsp");
}
When the user clicked on "Logout" button
//When the logout button is clicked if(request.getParameter("logout") != null)
{
//Invalidate the session
. session.invalidate();
if(cookies != null)
{
for(Cookie cookie : cookies)
{
//Capture the cookie "mrz" which we created when the user signing in
if(cookie.getName().equals("mrz"))
{
cookie.setMaxAge(0); //Make the cookie expired
cookie.setValue(null); //Unset the value of cookie
cookie.setPath("/");
response.addCookie(cookie); //Replace the cookie to save the changes
}
}
}
//Redirect the user to the login page
response.sendRedirect("login.jsp");
}
%>